Linked List
- Linked List can be defined as collection of objects called nodes that are randomly stored in the memory.
- A node contains two fields i.e. data stored at that particular address and the pointer which contains the address of the next node in the memory.
- The last node of the list contains pointer to the null.

Uses of Linked List
- The list is not required to be contiguously present in the memory. The node can reside any where in the memory and linked together to make a list. This achieves optimized utilization of space.
- list size is limited to the memory size and doesn't need to be declared in advance.
- Empty node can not be present in the linked list.
- We can store values of primitive types or objects in the singly linked list.
Why use linked list over array?
Till now, we were using array data structure to organize the group of elements that are to be stored individually in the memory. However, Array has several advantages and disadvantages which must be known in order to decide the data structure which will be used throughout the program.
Array contains following limitations:
- The size of array must be known in advance before using it in the program.
- Increasing size of the array is a time taking process. It is almost impossible to expand the size of the array at run time.
- All the elements in the array need to be contiguously stored in the memory. Inserting any element in the array needs shifting of all its predecessors.
Linked list is the data structure which can overcome all the limitations of an array. Using linked list is useful because,
- It allocates the memory dynamically. All the nodes of linked list are non-contiguously stored in the memory and linked together with the help of pointers.
- Sizing is no longer a problem since we do not need to define its size at the time of declaration. List grows as per the program's demand and limited to the available memory space.
Singly linked list or One way chain
Singly linked list can be defined as the collection of ordered set of elements. The number of elements may vary according to need of the program. A node in the singly linked list consist of two parts: data part and link part. Data part of the node stores actual information that is to be represented by the node while the link part of the node stores the address of its immediate successor.
One way chain or singly linked list can be traversed only in one direction. In other words, we can say that each node contains only next pointer, therefore we can not traverse the list in the reverse direction.
Consider an example where the marks obtained by the student in three subjects are stored in a linked list as shown in the figure.

In the above figure, the arrow represents the links. The data part of every node contains the marks obtained by the student in the different subject. The last node in the list is identified by the null pointer which is present in the address part of the last node. We can have as many elements we require, in the data part of the list.
Complexity
Data Structure | Time Complexity | Space Compleity | |||||||
---|---|---|---|---|---|---|---|---|---|
Average | Worst | Worst | |||||||
Access | Search | Insertion | Deletion | Access | Search | Insertion | Deletion | ||
Singly Linked List | θ(n) | θ(n) | θ(1) | θ(1) | O(n) | O(n) | O(1) | O(1) | O(n) |
Operations on Singly Linked List
There are various operations which can be performed on singly linked list. A list of all such operations is given below.
Node Creation
Insertion
The insertion into a singly linked list can be performed at different positions. Based on the position of the new node being inserted, the insertion is categorized into the following categories.
SN | Operation | Description |
---|---|---|
1 | Insertion at beginning | It involves inserting any element at the front of the list. We just need to a few link adjustments to make the new node as the head of the list. |
2 | Insertion at end of the list | It involves insertion at the last of the linked list. The new node can be inserted as the only node in the list or it can be inserted as the last one. Different logics are implemented in each scenario. |
3 | Insertion after specified node | It involves insertion after the specified node of the linked list. We need to skip the desired number of nodes in order to reach the node after which the new node will be inserted. . |
Insertion in singly linked list at beginning
Inserting a new element into a singly linked list at beginning is quite simple. We just need to make a few adjustments in the node links. There are the following steps which need to be followed in order to inser a new node in the list at beginning.
- Allocate the space for the new node and store data into the data part of the node. This will be done by the following statements.
- Make the link part of the new node pointing to the existing first node of the list. This will be done by using the following statement.
- At the last, we need to make the new node as the first node of the list this will be done by using the following statement.
Algorithm
- Step 1: IF PTR = NULL
Write OVERFLOW
Go to Step 7
[END OF IF]
- Step 2: SET NEW_NODE = PTR
- Step 3: SET PTR = PTR → NEXT
- Step 4: SET NEW_NODE → DATA = VAL
- Step 5: SET NEW_NODE → NEXT = HEAD
- Step 6: SET HEAD = NEW_NODE
- Step 7: EXIT
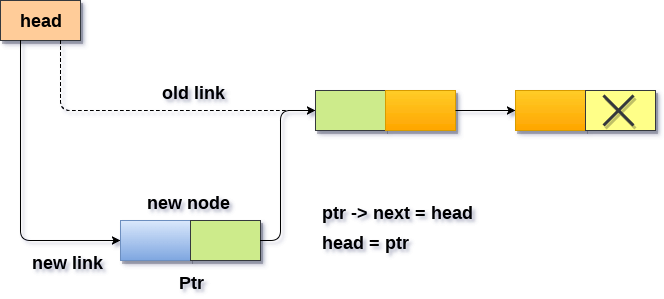
Write OVERFLOW
Go to Step 7
[END OF IF]
C Function
Output
Enter the item which you want to insert? 12 Node inserted Press 0 to insert more ? 0 Enter the item which you want to insert? 23 Node inserted Press 0 to insert more ? 2
Insertion in singly linked list at the end
In order to insert a node at the last, there are two following scenarios which need to be mentioned.
- The node is being added to an empty list
- The node is being added to the end of the linked list
In order to insert a node at the last, there are two following scenarios which need to be mentioned.
- The node is being added to an empty list
- The node is being added to the end of the linked list
in the first case,
- The condition (head == NULL) gets satisfied. Hence, we just need to allocate the space for the node by using malloc statement in C. Data and the link part of the node are set up by using the following statements.
- Since, ptr is the only node that will be inserted in the list hence, we need to make this node pointed by the head pointer of the list. This will be done by using the following Statements.
In the second case,
- The condition Head = NULL would fail, since Head is not null. Now, we need to declare a temporary pointer temp in order to traverse through the list. temp is made to point the first node of the list.
- Then, traverse through the entire linked list using the statements:
- At the end of the loop, the temp will be pointing to the last node of the list. Now, allocate the space for the new node, and assign the item to its data part. Since, the new node is going to be the last node of the list hence, the next part of this node needs to be pointing to the null. We need to make the next part of the temp node (which is currently the last node of the list) point to the new node (ptr) .
Algorithm
- Step 1: IF PTR = NULL Write OVERFLOW
Go to Step 1
[END OF IF] - Step 2: SET NEW_NODE = PTR
- Step 3: SET PTR = PTR - > NEXT
- Step 4: SET NEW_NODE - > DATA = VAL
- Step 5: SET NEW_NODE - > NEXT = NULL
- Step 6: SET PTR = HEAD
- Step 7: Repeat Step 8 while PTR - > NEXT != NULL
- Step 8: SET PTR = PTR - > NEXT
[END OF LOOP] - Step 9: SET PTR - > NEXT = NEW_NODE
- Step 10: EXIT
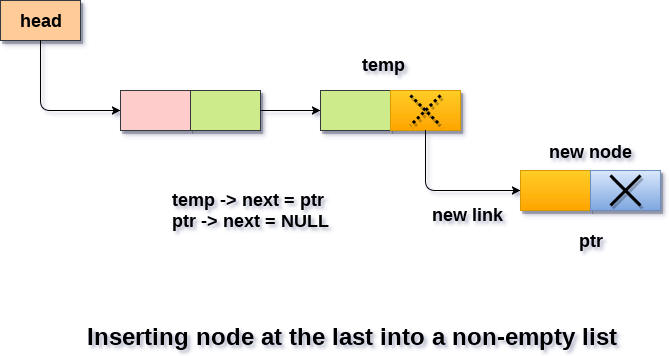
Go to Step 1
[END OF IF]
[END OF LOOP]
C Function
Output
Enter the item which you want to insert?
12
Node inserted
Press 0 to insert more ?
0
Enter the item which you want to insert?
23
Node inserted
Press 0 to insert more ?
2
Output
Enter the item which you want to insert? 12 Node inserted Press 0 to insert more ? 0 Enter the item which you want to insert? 23 Node inserted Press 0 to insert more ? 2
Insertion in singly linked list after specified Node
- In order to insert an element after the specified number of nodes into the linked list, we need to skip the desired number of elements in the list to move the pointer at the position after which the node will be inserted. This will be done by using the following statements.
- Allocate the space for the new node and add the item to the data part of it. This will be done by using the following statements.
- Now, we just need to make a few more link adjustments and our node at will be inserted at the specified position. Since, at the end of the loop, the loop pointer temp would be pointing to the node after which the new node will be inserted. Therefore, the next part of the new node ptr must contain the address of the next part of the temp (since, ptr will be in between temp and the next of the temp). This will be done by using the following statements.
now, we just need to make the next part of the temp, point to the new node ptr. This will insert the new node ptr, at the specified position.
Algorithm
- STEP 1: IF PTR = NULL
WRITE OVERFLOW
GOTO STEP 12
END OF IF
- STEP 2: SET NEW_NODE = PTR
- STEP 3: NEW_NODE → DATA = VAL
- STEP 4: SET TEMP = HEAD
- STEP 5: SET I = 0
- STEP 6: REPEAT STEP 5 AND 6 UNTIL I
- STEP 7: TEMP = TEMP → NEXT
- STEP 8: IF TEMP = NULL
WRITE "DESIRED NODE NOT PRESENT"
GOTO STEP 12
END OF IF
END OF LOOP
- STEP 9: PTR → NEXT = TEMP → NEXT
- STEP 10: TEMP → NEXT = PTR
- STEP 11: SET PTR = NEW_NODE
- STEP 12: EXIT
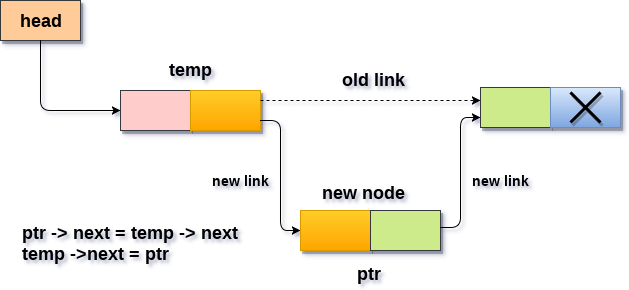
WRITE OVERFLOW
GOTO STEP 12
END OF IF
WRITE "DESIRED NODE NOT PRESENT"
GOTO STEP 12
END OF IF
END OF LOOP
C Function
Output
Enter the item which you want to insert? 12 Node inserted Press 0 to insert more ? 2
Deletion and Traversing
The Deletion of a node from a singly linked list can be performed at different positions. Based on the position of the node being deleted, the operation is categorized into the following categories.
SN | Operation | Description |
---|---|---|
1 | Deletion at beginning | It involves deletion of a node from the beginning of the list. This is the simplest operation among all. It just need a few adjustments in the node pointers. |
2 | Deletion at the end of the list | It involves deleting the last node of the list. The list can either be empty or full. Different logic is implemented for the different scenarios. |
3 | Deletion after specified node | It involves deleting the node after the specified node in the list. we need to skip the desired number of nodes to reach the node after which the node will be deleted. This requires traversing through the list. |
4 | Traversing | In traversing, we simply visit each node of the list at least once in order to perform some specific operation on it, for example, printing data part of each node present in the list. |
5 | Searching | In searching, we match each element of the list with the given element. If the element is found on any of the location then location of that element is returned otherwise null is returned. . |
Deletion in singly linked list at beginning
Deleting a node from the beginning of the list is the simplest operation of all. It just need a few adjustments in the node pointers. Since the first node of the list is to be deleted, therefore, we just need to make the head, point to the next of the head. This will be done by using the following statements.
Now, free the pointer ptr which was pointing to the head node of the list. This will be done by using the following statement.
Algorithm
- Step 1: IF HEAD = NULL
Write UNDERFLOW
Go to Step 5
[END OF IF]
- Step 2: SET PTR = HEAD
- Step 3: SET HEAD = HEAD -> NEXT
- Step 4: FREE PTR
- Step 5: EXIT
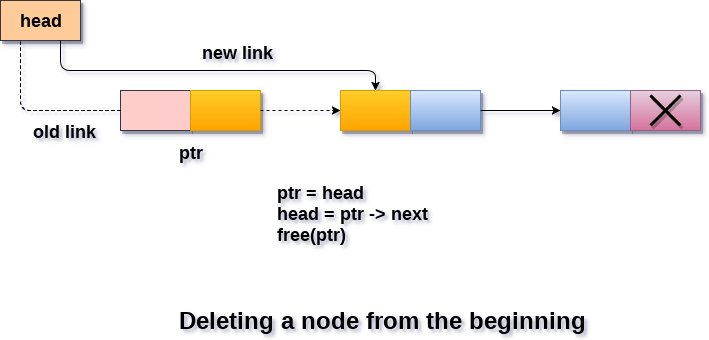
Write UNDERFLOW
Go to Step 5
[END OF IF]
C function
Output
1.Append List 2.Delete node 3.Exit 4.Enter your choice?1 Enter the item 23 Node inserted 1.Append List 2.Delete node 3.Exit 4.Enter your choice?2 Node deleted from the begining ...
Deletion in singly linked list at the end
There are two scenarios in which, a node is deleted from the end of the linked list.
- There is only one node in the list and that needs to be deleted.
- There are more than one node in the list and the last node of the list will be deleted.
In the first scenario,
the condition head → next = NULL will survive and therefore, the only node head of the list will be assigned to null. This will be done by using the following statements.
In the second scenario,
The condition head → next = NULL would fail and therefore, we have to traverse the node in order to reach the last node of the list.
For this purpose, just declare a temporary pointer temp and assign it to head of the list. We also need to keep track of the second last node of the list. For this purpose, two pointers ptr and ptr1 will be used where ptr will point to the last node and ptr1 will point to the second last node of the list.
this all will be done by using the following statements.
Now, we just need to make the pointer ptr1 point to the NULL and the last node of the list that is pointed by ptr will become free. It will be done by using the following statements.
Algorithm
- Step 1: IF HEAD = NULL
Write UNDERFLOW
Go to Step 8
[END OF IF]
- Step 2: SET PTR = HEAD
- Step 3: Repeat Steps 4 and 5 while PTR -> NEXT!= NULL
- Step 4: SET PREPTR = PTR
- Step 5: SET PTR = PTR -> NEXT
[END OF LOOP]
- Step 6: SET PREPTR -> NEXT = NULL
- Step 7: FREE PTR
- Step 8: EXIT
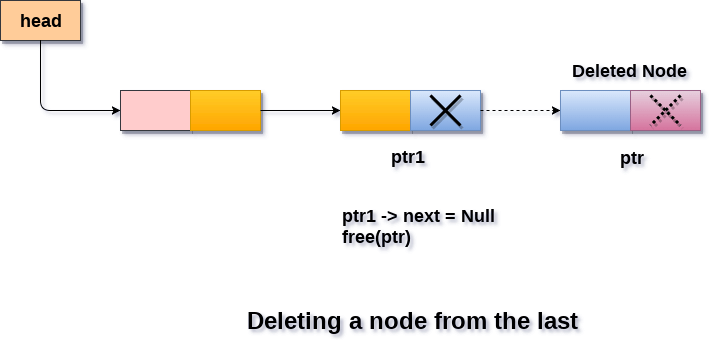
Write UNDERFLOW
Go to Step 8
[END OF IF]
[END OF LOOP]
C Function :
Output
1.Append List 2.Delete node 3.Exit 4.Enter your choice?1 Enter the item 12 Node inserted 1.Append List 2.Delete node 3.Exit 4.Enter your choice?2 Only node of the list deleted ...
Deletion in singly linked list after the specified node :
In order to delete the node, which is present after the specified node, we need to skip the desired number of nodes to reach the node after which the node will be deleted. We need to keep track of the two nodes. The one which is to be deleted the other one if the node which is present before that node. For this purpose, two pointers are used: ptr and ptr1.
Use the following statements to do so.
Now, our task is almost done, we just need to make a few pointer adjustments. Make the next of ptr1 (points to the specified node) point to the next of ptr (the node which is to be deleted).
This will be done by using the following statements.
Algorithm
- STEP 1: IF HEAD = NULL
WRITE UNDERFLOW
GOTO STEP 10
END OF IF
- STEP 2: SET TEMP = HEAD
- STEP 3: SET I = 0
- STEP 4: REPEAT STEP 5 TO 8 UNTIL I
- STEP 5: TEMP1 = TEMP
- STEP 6: TEMP = TEMP → NEXT
- STEP 7: IF TEMP = NULL
WRITE "DESIRED NODE NOT PRESENT"
GOTO STEP 12
END OF IF
- STEP 8: I = I+1
END OF LOOP
- STEP 9: TEMP1 → NEXT = TEMP → NEXT
- STEP 10: FREE TEMP
- STEP 11: EXIT

WRITE UNDERFLOW
GOTO STEP 10
END OF IF
WRITE "DESIRED NODE NOT PRESENT"
GOTO STEP 12
END OF IF
END OF LOOP
C function
Output
1.Append List 2.Delete node 3.Exit 4.Enter your choice?1 Enter the item 12 Node inserted 1.Append List 2.Delete node 3.Exit 4.Enter your choice?1 Enter the item 23 Node inserted 1.Append List 2.Delete node 3.Exit 4.Enter your choice?2 12 There are less than 12 elements in the list.. 1.Append List 2.Delete node 3.Exit 4.Enter your choice?2 1 Deleted 1 node
Traversing in singly linked list
Traversing is the most common operation that is performed in almost every scenario of singly linked list. Traversing means visiting each node of the list once in order to perform some operation on that. This will be done by using the following statements.
Algorithm
- STEP 1: SET PTR = HEAD
- STEP 2: IF PTR = NULL
WRITE "EMPTY LIST"
GOTO STEP 7
END OF IF
- STEP 4: REPEAT STEP 5 AND 6 UNTIL PTR != NULL
- STEP 5: PRINT PTR→ DATA
- STEP 6: PTR = PTR → NEXT
[END OF LOOP]
- STEP 7: EXIT
WRITE "EMPTY LIST"
GOTO STEP 7
END OF IF
[END OF LOOP]
C function
Output
1.Append List 2.Traverse 3.Exit 4.Enter your choice?1 Enter the item 23 Node inserted 1.Append List 2.Traverse 3.Exit 4.Enter your choice?1 Enter the item 233 Node inserted 1.Append List 2.Traverse 3.Exit 4.Enter your choice?2 printing values . . . . . 233 23
Searching in singly linked list
Searching is performed in order to find the location of a particular element in the list. Searching any element in the list needs traversing through the list and make the comparison of every element of the list with the specified element. If the element is matched with any of the list element then the location of the element is returned from the function.
Algorithm
- Step 1: SET PTR = HEAD
- Step 2: Set I = 0
- STEP 3: IF PTR = NULL
WRITE "EMPTY LIST"
GOTO STEP 8
END OF IF
- STEP 4: REPEAT STEP 5 TO 7 UNTIL PTR != NULL
- STEP 5: if ptr → data = item
write i+1
End of IF
- STEP 6: I = I + 1
- STEP 7: PTR = PTR → NEXT
[END OF LOOP]
- STEP 8: EXIT
WRITE "EMPTY LIST"
GOTO STEP 8
END OF IF
write i+1
End of IF
[END OF LOOP]
C function
Output
1.Create 2.Search 3.Exit 4.Enter your choice?1 Enter the item 23 Node inserted 1.Create 2.Search 3.Exit 4.Enter your choice?1 Enter the item 34 Node inserted 1.Create 2.Search 3.Exit 4.Enter your choice?2 Enter item which you want to search? 34 item found at location 1
Linked List in C: Menu Driven Program
Output:
*********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 1 Enter value 1 Node inserted *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 2 Enter value? 2 Node inserted *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 3 Enter element value1 Enter the location after which you want to insert 1 Node inserted *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 8 printing values . . . . . 1 2 1 *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 2 Enter value? 123 Node inserted *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 1 Enter value 1234 Node inserted *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 4 Node deleted from the begining ... *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 5 Deleted Node from the last ... *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 6 Enter the location of the node after which you want to perform deletion 1 Deleted node 2 *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 8 printing values . . . . . 1 1 *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 7 Enter item which you want to search? 1 item found at location 1 item found at location 2 *********Main Menu********* Choose one option from the following list ... =============================================== 1.Insert in begining 2.Insert at last 3.Insert at any random location 4.Delete from Beginning 5.Delete from last 6.Delete node after specified location 7.Search for an element 8.Show 9.Exit Enter your choice? 9
0 Comments